Quite often I want to be able to hover over a type to see it’s full type but VSCode truncates it. This is makes it really annoying to look for properties and their underlying types.
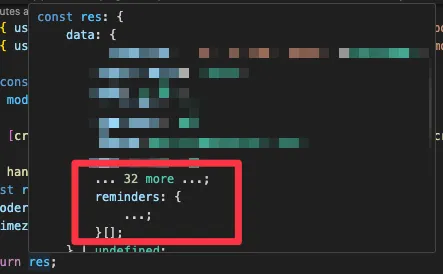
It turns out, the Typescript server has a property called defaultMaximumTruncationLength
which by default sets the maximum type hint length to 160.
It’s a bit fiddly to change this value so I wrote a script (or rather, patched together a load of scripts other people have written) which allows me to change this value on the fly. This is handy because TS truncates these hints to avoid overloading your computer. By being able to set it on the fly, you can revert it back afterwards to avoid these performance issues.
Here is the script:
change_ts_hint_length() { # Add your VScode path here directory_path="/Applications/Visual Studio Code.app" new_length="$1"
if [ -z "$1" ] then echo "No length supplied, will use default (160)" new_length=160 fi
file_path=$(find "$directory_path" -name "tsserver.js" 2>/dev/null) if [ -z "$file_path" ]; then echo "tsserver.js file not found within directory $directory_path after recursive search" exit 1 fi
# echo "Found tsserver.js at \"$file_path\""
# Use awk to perform the replacement awk -v new_length="$new_length" '{sub(/var defaultMaximumTruncationLength = [0-9]+;/, "var defaultMaximumTruncationLength = " new_length ";")}1' "$file_path" > temp_file && mv temp_file "$file_path"
echo "Length updated successfully: " cat "$file_path" | grep "defaultMaximumTruncationLength = "
}
alias tslen="change_ts_hint_length"
I added this to my zshprofile and as you can see I assigned it an alias of tslen
(don’t forget to add the path to your VSCode installation as it says on the top line)
Now, when I want to hover over a truncated type, I do the following:
tslen 10000
Then I run cmd
+ shift
+ p
to open the VSCode command palette and run the “Typescript: Restart TS Server” command. Once this is done, hovering over the type will show the full type (assuming it is smaller than the length I passed in).
Once I am done, I revert the variable by repeating the process but this time I don’t pass in an argument (in which case the script reverts it to the default length of 160)